3.1.1.2 BLE Legacy Scan
Getting Started with Central Building Blocks
Introduction
This document helps users to enable the BLE Scanning on WBZ451 Curiosity board using MPLAB Code Configurator(MCC). BLE Scanning is for devices that are advertising. In BLE, a central or observer always starts with scanning.
Users of this document can choose to just run the precompiled Application Example hex file on the WBZ451 Curiosity Board and experience the demo or can go through the steps involved in developing this application from scratch
It is recommend to follow the examples in order, by learning the basic concepts first and then progressing to the more advanced topics..
Recommended Reading
Hardware Requirement
Tool | Qty |
---|---|
WBZ451 Curiosity Board | 1 |
Micro USB cable | 1 |
SDK Setup
Software
Smartphone App
None
Programming the Precompiled hex File or Application Example
Programming the hex file using MPLABX IPE
-
Precompiled Hex file is located in "<Harmony Content Path>\wireless_apps_pic32cxbz2_wbz45\apps\ble\building_blocks\central\legacy_scan\hex" folder
-
Follow the steps mentioned hereNote: Users must choose the correct Device and Tool information.
Programming the Application using MPLABX IDE
-
Follow steps mentioned in of Running a Precompiled Example document.
-
Open and program the Application Example "legacy_scan.x" located in "<Harmony Content Path>\wireless_apps_pic32cxbz2_wbz45\apps\ble\building_blocks\central\legacy_scan\firmware" using MPLABX IDE
For more details on how to find the Harmony Content Path, refer to Installing the MCC Plugin
Demo Description
This Application Example enables users to do passive scanning. After programming the Application Example, on reset user will be able to see the beginning of scan operation, the Bluetooth addresses of devices scanned for the next 10 seconds, the end of scanning operation
Testing
Connect the WBZ451 Curiosity board to PC, program the precompiled hex file or application example as mentioned. Open TeraTerm @ (Speed: 115200, Data: 8-bit, Parity: none, stop bits: 1 bit, Flow control: none). Reset the board. Upon reset, "Scanning" message is displayed on the TeraTerm. Once scan operation has begun user will be able to display all the Bluetooth addresses that are advertising on channel 37, 38 and 39. After 10 secs "Scan Completed" message is sent out.
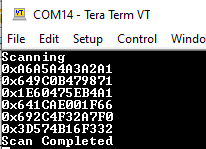
Users can use another WBZ451 Curiosity Board configured as BLE Advertiser set the address and scan
Developing this Application from scratch using MPLAB Code Configurator
-
Create a new MCC Harmony Project. For more details, refer to 2.5 Creating a New MCC Harmony Project.
Import component configuration: This step helps users setup the basic components and configuration required to develop this application. The imported file is of format .mc3 and is located in the path
"<Harmony Content Path>\wireless_apps_pic32cxbz2_wbz45\apps\ble\building_blocks\central\legacy_scan\firmware\legacy_scan.X".
For more details on how to import the component configuration , refer to14.3 Importing Existing App Example Configuration
Accept Dependencies or satisfiers, select Yes
- Verify if the Project Graph window has all the expected configuration
Verifying the Scan Configuration
- Select BLE_Stack component in project graph
Generating a Code
For more details on code generation, refer to 14.2 MPLAB Code Configurator(MCC) Code Generation.
Files and Routines Automatically generated by the MCC
The BLE stack initialization routine executed during Application Initialization can be found in project files. This intitialization routine is automatically generated by the MCC. This call initializes and configures the GAP, GATT, SMP, L2CAP and BLE middleware layers.
Autogenerated, Scan Parameters

Source Files | Usage |
---|---|
app.c | Application State machine, includes calls for Initialization of all BLE stack (GAP,GATT, SMP, L2CAP) related component configurations |
app_ble\app_ble.c | Source Code for the BLE stack related component configurations, code related to function calls from app.c |
app_ble\ app_ble_handler.c | All GAP, GATT, SMP and L2CAP Event handlers |
Note: app.c is autogenerated and has a state machine based Application code sample, users can use this template to develop their application |
Header Files
ble_gap.h-
This header file contains BLE GAP functions and is automatically included in the app.c file
Function Calls
MCC generates and adds the code to initialize the BLE Stack GAP, GATT, L2CAP and SMP in APP_BleStackInit() function
APP_BleStackInit() is the API that will be called inside the Applications Initial State -- APP_STATE_INIT in app.c
User Application Development
Include
User action is required as mentioned here
definitions.h
in all the files where UART will be used to print debug informationNote:definitions.h
is not specific to just UART peripheral, instead it must be included in all application source files where peripheral functionality will be exercised
Start Scanning
BLE_GAP_SetScanningEnable(true, BLE_GAP_SCAN_FD_ENABLE, BLE_GAP_SCAN_MODE_OBSERVER, 100);
Scan Results
BLE_GAP_EVT_ADV_REPORT event is generated upon finding Adverstisements on legacy channels
// code snippet to print scan results
uint8_t scanAddr[12]; //var to store ASCII address
BLE_GAP_EvtAdvReport_T scanResults;
scanResults.addr = p_event->eventField.evtAdvReport.addr;
APP_HexToAscii(6, scanResults.addr.addr, scanAddr);
SERCOM0_USART_Write((uint8_t *)"0x", 2);
SERCOM0_USART_Write((uint8_t *)scanAddr, 12);
SERCOM0_USART_Write((uint8_t *)"\r\n", 2);
// function to convert HEX to ASCII
static void APP_HexToAscii(uint8_t byteNum, uint8_t *p_hex, uint8_t *p_ascii)
{
uint8_t i, j, c;
uint8_t digitNum = byteNum * 2;
if (p_hex == NULL || p_ascii == NULL)
return;
for (i = 0; i < digitNum; i++)
{
j = i / 2;
c = p_hex[j] & 0x0F;
if (c >= 0x00 && c <= 0x09)
{
p_ascii[digitNum - i - 1] = c + 0x30;
}
else if (c >= 0x0A && c <= 0x0F)
{
p_ascii[digitNum - i - 1] = c - 0x0A + 'A';
}
p_hex[j] /= 16;
}
}
Scan Timeout Event
- In app_ble_handler.c BLE_GAP_EVT_SCAN_TIMEOUT event is generated when BLE Scan duration expires.
Users can exercise various other BLE Advertisement functionalities by using BLE Stack API
For more details, refer to BLE Connection.